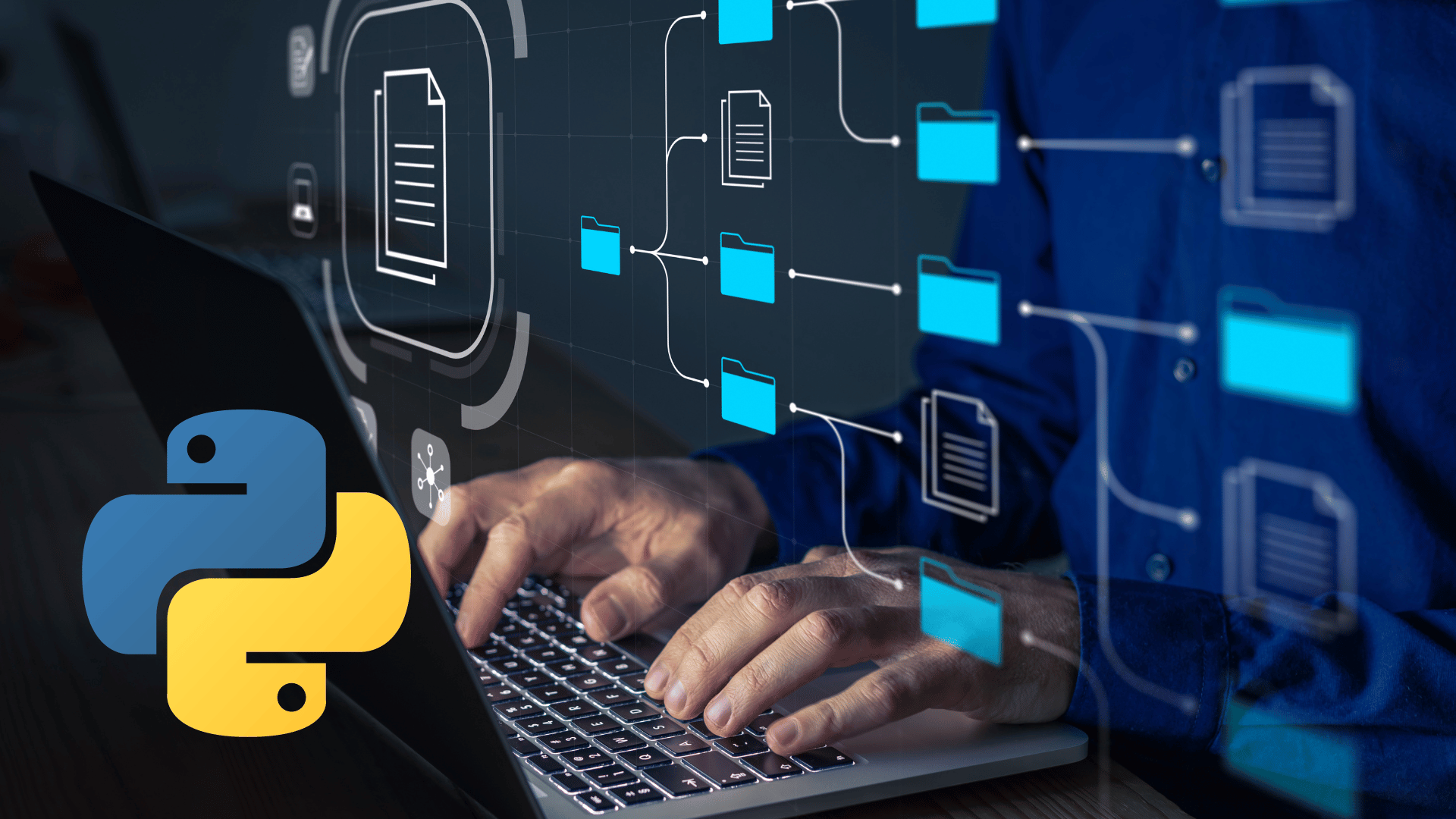
Python, one of the most versatile and scalable programming languages, offers a multitude of libraries, each designed to make a coder's life easier. Among them, we often overlook one that serves a crucial purpose - file and directory management. Traditional file handling methods, though practical, oftentimes lack readability and convenience.
Imagine streamlining your file management tasks in Python - creating, reading, writing files, and navigating directories - efficiently and in a more 'pythonic' way. Seems fantastic, doesn't it? Welcome to the world of pathlib
- an object-oriented library designed to handle filesystem paths.
In this blog, we'll dive into pathlib
's fascinating world and get comfortable shifting our file operations to this modern, robust library. Ready to boost your Python skills to the next level? Join us on this exciting journey as we explore the many facets of pathlib
, master its methods, and wield it proficiently in our programming tasks.
Introduction to pathlib
Gone are the days when handling filesystem paths was clumsy and error-prone. Thanks to Python's pathlib
module, a library that is part of Python's standard library, we now have a cleaner and more streamlined approach to deal with files and directories.
In essence, pathlib
is a paradigm shift in how we work with file paths. It bundles most of the functionalities of os
, os.path
, and glob
into one simple and attractive API. Think of pathlib
as an all-in-one toolbox for anything path-related.
pathlib
provides classes for pure path objects that implement operations on strings representing filesystem paths, independently of whether those paths are supported or exist physically on the disk. These are called pure paths.
On the other hand, concrete path objects that provide methods to do system calls on filesystem paths are variants of the Path
class. Pure paths are divided into PurePosixPath
and PureWindowsPath
classes, while concrete paths are represented by PosixPath
and WindowsPath
classes.
Pure Paths
PurePath
objects provide path-handling operations which don't interact with the file system. We use subclasses PurePosixPath
and PureWindowsPath
depending on the system under use.
pythonfrom pathlib import PurePath p = PurePath('sample/path') print(p)
Output:
shellsample/path
PurePosixPath
If you're on Unix/Linux, you use PurePosixPath
.
pythonfrom pathlib import PurePosixPath pp = PurePosixPath('example/path') print(pp)
Output:
shellexample/path
PureWindowsPath
On the other hand, if you're on Windows, you'd use PureWindowsPath
.
pythonfrom pathlib import PureWindowsPath pw = PureWindowsPath('example\\path') print(pw)
Output:
shellexample\path
Path
Path
objects are general and can become whatever their system paths are. Therefore, if you're coding on a Windows system, Path
becomes WindowsPath
, while it becomes PosixPath
on a Unix-based system.
pythonfrom pathlib import Path p = Path('sample/path') print(p)
If you run this on Unix/Linux, the output will be PosixPath('sample/path')
. On Windows, it'll be WindowsPath('sample\\path')
.
The Differences
PurePath
objects are very handy when you want to manipulate paths and extract information from them purely through string manipulation, whereas Path
objects actually interact with the file system, allowing operations like checking a file's size or reading a file's content.
I hope this clears up the use and nature of PurePath
and Path
. Now you can choose which one fits your needs better in your Python file handling journey!
-
The
Path
objects, often referred to as concrete paths, can do everything thePurePath
can and much more. Their power, however, comes with a limitation; they are not available in all operating systems. -
The
PurePath
objects, thus, allow us to work with file paths, with methods that work everywhere. They are often referred to as pure paths - file paths that make no system calls.
Getting Started with pathlib
Working with pathlib
is quite intuitive and looks more like natural language, making your code easier to understand. Let's delve deeper into its features.
Installation
For Python 3.4 and above, pathlib
is a built-in module, so you don't need to install anything. If you're below Python 3.4, consider updating Python, or install the pathlib
library via pip
:
shellpip install pathlib
Importing pathlib
To work with pathlib
, we need to import it into our Python environment.
pythonfrom pathlib import Path
Creating a Path
With pathlib
, creating a new path becomes straightforward.
pythonp = Path('.') print(p)
Output:
shell.
Here, the "." refers to the current directory.
Reading and Writing Files
You can use pathlib
to open a file, read from it or write to it. Let's create a text file and write some text into it:
pythonp = Path('testfile.txt') p.write_text('Hello, Pythonista!') print(p.read_text())
Output:
shellHello, Pythonista!
Understanding Absolute and Relative Paths
An absolute path refers to the complete details needed to locate a file or folder, starting from the root element and ending with the other subdirectories.
A relative path, on the other hand, is relative to the program’s current working directory.
To get an absolute path using pathlib
:
pythonp = Path('.') print(p.resolve())
Output will show the complete path to your current directory.
Navigating Directories
To navigate to a parent directory:
pythonp = Path('/usr/bin/python3') print(p.parent)
Output:
shell/usr/bin
To navigate to a child directory:
pythonp = Path('.') q = p / 'child_directory' print(q)
Output:
shell./child_directory
pathlib
makes it incredibly easy to create, write, read, and navigate files and directories. Let's move forward and unlock more of this powerful library's potential!
Advanced Concepts in pathlib
Armed with the basics, let's explore some advanced functionalities that pathlib
offers.
Check File and Directory Existence
How about checking if a certain file or path already exists? With pathlib
, it's simple!
pythonp = Path('testfile.txt') print(p.exists())
This will output True
if the file testfile.txt
exists, and False
otherwise.
Check if it's a File or Directory
Sometimes, you might need to ensure if a particular path is a file or a directory. This can easily be achieved using the is_dir()
and is_file()
methods.
pythonp = Path('testfile.txt') print(p.is_file())
This will return True
if testfile.txt
is a file. Similarly, you can check if a path is a directory:
pythonp = Path('/usr/bin') print(p.is_dir())
Working with Glob Pattern
The glob()
method is extremely handy in matching pathnames. It supports the *
, ?
, and []
pattern matching similar to a Unix shell.
pythonp = Path('.') for file in p.glob('*'): print(file)
This code will print all the files and directories in the current directory.
Manipulating File Attributes
pathlib
can access many kinds of file and directory details, which include permissions, size, and date of creation. Below is an example of obtaining file size:
pythonp = Path('testfile.txt') print(p.stat().st_size)
This will print the size of testfile.txt
in bytes.
Renaming and Deleting Files
Renaming and deleting files is seamless with pathlib
.
To rename a file:
pythonp = Path('old_name.txt') p.rename('new_name.txt')
This will rename the file old_name.txt
to new_name.txt
.
To delete a file:
pythonp = Path('somefile.txt') p.unlink()
This will delete the file somefile.txt
.
pathlib
not only simplifies file and directory handling in Python, but also makes it more robust and reliable. In the next section, let's look at real scenarios where pathlib
can be leveraged.
Use Cases & Examples
pathlib
is not just about convenience, it is a power-tool that enables more efficient file handling operations in various scenarios. Here are some practical use cases and step-by-step examples of how pathlib
makes programming a breeze.
Use Case 1: Creating Directories for a New Project
Let's imagine you are starting a new Python project and you need to create a directory structure. pathlib
makes this straightforward.
pythonp = Path('/your/desired/directory') p.mkdir(parents=True, exist_ok=True)
This will create all directories in the specified path if they don't exist. parents=True
makes sure all parent directories are created if they don't exist and exist_ok=True
prevents any error if the directories already exist.
Use Case 2: Finding Specific Files
Need to find all Python files in a directory? Use glob()
method!
pythonp = Path('.') for python_file in p.glob('*.py'): print(python_file)
This script will print all the Python (.py) files in the current directory.
Use Case 3: Making a Backup of a File
Backing up a file becomes a simple operation with pathlib
. Let's say we wish to make a backup of testfile.txt
.
pythonp = Path('testfile.txt') p.rename('testfile_backup.txt')
This script will rename testfile.txt
to testfile_backup.txt
.
Use Case 4: Joining Paths
pathlib
makes joining paths together extremely intuitive, handling all the complexities of slashes and double slashes.
pythonp = Path('/usr') q = p / 'bin' print(q)
This script will print /usr/bin
, thereby joining bin
to the directory /usr
cleverly.
Use Case 5: Iterating Through a Directory and its Subdirectories
You might need to list all files in a directory and its subdirectories:
pythonfor file in Path('.').rglob('*'): print(file)
This script uses rglob()
, a variant of glob()
to recursively print all files and directories from the current directory and its subdirectories.
That's it! You've now seen firsthand how pathlib
can streamline your file handling tasks! In the next section, we'll wrap up everything we've learned about this impressive library. Let's keep Pythoning with pathlib
!
Conclusion
From simplifying the creation of directories to managing files on your system, pathlib
truly offers a high-level, intuitive approach to the filesystem. Hopefully, this blog acquainted you with its power and versatile usage. Remember, no matter how complex the problem might look, Python, especially with tools like pathlib
, can simplify it to a breeze. So, grab your cup of coffee, and keep coding!