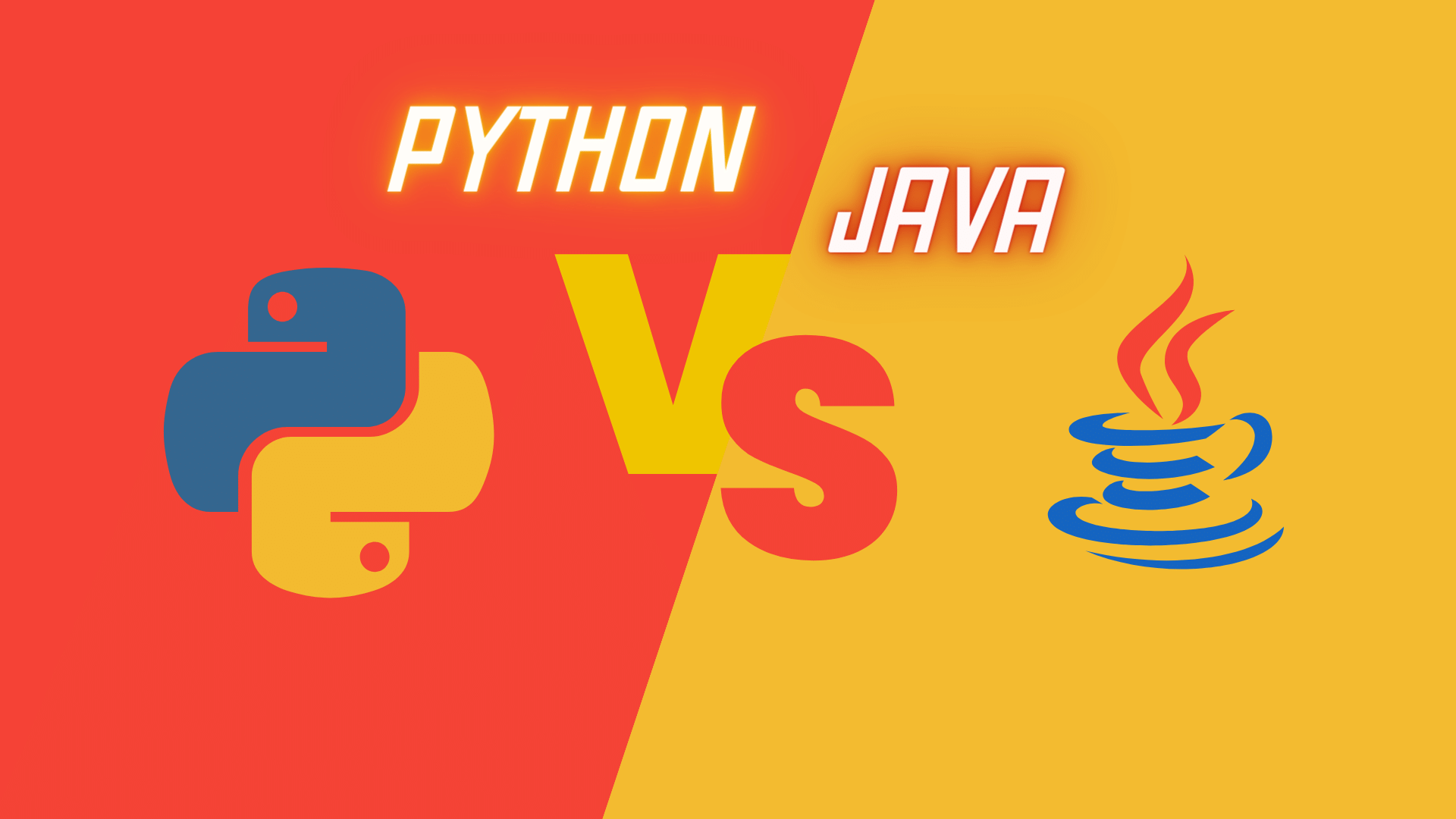
CONTENTS
If you're a Java developer curious about learning Python, you've come to the right place. This article will navigate you through Python's key aspects using your existing knowledge of Java to make the journey easier. We will leverage simple language and illustrative examples to help you grasp Python quickly and effectively.
The Basics
Before delving into in-depth comparisons, let's understand the basic layout and features of both languages:
-
Object-Oriented Programming: Both Java and Python adopt object-oriented programming, which means they both support the concept of classes, inheritance, encapsulation, and polymorphism.
-
Interpreted vs Compiled: Java is a compiled language where the source code is transformed into bytecode, and then the JVM executes this bytecode. Python, however, is an interpreted language, which means the Python interpreter reads and executes the code line by line.
-
Type System: Java is statically-typed, meaning you must declare the type of a variable when you create it, and the variable type cannot be changed later. On the other hand, Python is dynamically-typed, allowing you to alter a variable's type at runtime.
-
Syntactical Differences: One of the significant differences between Java and Python is in their syntax. Java requires semicolons to end statements and braces to define a block of code. Python uses indentation to mark a block of code and does not need any statement terminator.Python, on the other hand:
- Does not use semicolons (;) to end statements.
- Does not require types to be declared.
- Uses indentation to denote a block of code instead of braces ().
- Does not use parentheses around expressions.
- Comments are written with the hashtag symbol (#).
python# simple_python_program.py numbers = [1, -10, 0, -5, -1000, 100, 7] maximum = numbers[0] for number in numbers: if number > maximum: maximum = number print("The maximum value is", maximum)
CPython vs the JIT Compiler
Python typically uses an interpreter called CPython as the default runtime environment, which interprets Python code into bytecode and then interprets that into machine code.
CPython, written in C, is not just an interpreter but also a compiler — it compiles Python code into intermediate bytecode then interprets this bytecode into machine code.
python# Python code print("Hello, world!")
This is first compiled
to bytecode. To a certain extent, this process is similar to the way javac
compiles a .java
file into a .class
file.
bytecode# Example Bytecode LOAD_GLOBAL 0 (print) LOAD_CONST 1 ('Hello, world!') CALL_FUNCTION 1 RETURN_VALUE
Then, at runtime, the bytecode is interpreted line by line
. CPython, unlike mainstream JVM implementations, doesn't compile the obtained bytecode into native object code.
Java, on the other hand, utilizes a Just-In-Time (JIT) compiler in the JVM (Java Virtual Machine). The JIT compiler transforms the bytecode into machine-level instructions so that it can be directly executed by the system's physical processor. The primary advantage of a JIT compiler over a standard compiler is that it can improve the runtime performance.
Note that there are several other Python implementations that adopt a different approach:
- Jython: This Python implementation is crafted for the Java platform. It runs within the JVM, with direct interoperability between Java and Python.
- IronPython: Similar to Jython, IronPython is an implementation that operates within the .NET platform.
- PyPy: PyPy employs a Just-In-Time (JIT) compiler and, on average, performs approximately 4.2 times faster than CPython.
- GraalVM: GraalVM is a superior runtime environment that supports a multitude of programming languages. It provides experimental support for rather recent Python versions.
Memory Management
Python and Java handle memory management quite differently.
In Java, memory management is done through the Java Virtual Machine (JVM). The JVM uses an automatic garbage collector to deallocate memory. It looks at heap memory, identifies which objects are in use (called live objects) and which are not, and deletes the unused objects. These garbage collection routines run in a separate high-priority thread in the background, ensuring the availability of maximum free heap space to the running Java application.
On the other hand, in Python, memory management is done through a reference counting for garbage collection. Python automatically counts the number of references to an object, and once an object's reference count drops to zero, it is deleted. Python also includes a cycle-detecting collector to manage reference cycles - situations where objects reference each other, and the reference count never drops to zero.
Variable Declaration
In Java, variable declaration involves specifying the variable type, followed by the variable name and value. Java is statically typed
, meaning the variable type has to be declared when it's initialized and cannot change afterwards.
javaString myVariable = "I am a Java variable";
In Python, the variable declaration is much simpler and doesn't require specifying the variable's type. Python is dynamically typed
, allowing you to change a variable's type during runtime.
pythonmy_variable = "I am a Python variable"
Key Differences
- Declaration Syntax: While Java requires variable type declarations, Python does not.
- Typing: Java is
statically typed
, while Python isdynamically typed
.
Note that Python is at the same time a strongly typed language:
- Every object has a specific type associated with it.
- Explicit conversion is needed between incompatible types.
python40 + "2" # TypeError: unsupported operand type(s) # for +: 'int' and 'str' 40 + int("2") # Add two numbers - Explicit converision
Data Types in Python and Java
Understanding the differences in fundamental data types is crucial when switching from Java to Python. In Java, we have eight primitive data types - byte, short, int, long, float, double, char, and boolean.
javabyte byteExample = 10; // Byte short shortExample = 500; // Short int intExample = 20000; // Int long longExample = 300000L; // Long float floatExample = 20.0f; // Float double doubleExample = 200.20d; // Double char charExample = 'J'; // Char boolean booleanExample = true; // Boolean
Python, on the other hand, simplifies things to a great extent. It only has four basic types: int, float, bool, and str
pythonintExample = 20000 # Integer floatExample = 200.20 # Float booleanExample = True # Boolean charExample = 'J' # String (Character) stringExample = 'Python' # Strings
Strings
In both Java and Python, strings are sequences of characters used for text storage and manipulation. Python offers different types of strings, such as byte strings, raw strings, and Unicode strings, which provide more flexibility and options to developers.
Java strings can be declared using double quotes:
javaString hello = "Hello World";
In Python also, strings can be declared either using single quotes ('
) or double quotes ("
), both of which have identical functionality.
pythonhello = 'Hello World' greeting = "Hello again!"
Python also allows declaring byte strings, Unicode strings, and raw strings:
pythonbyte_string = b"Hello Bytes" unicode_string = u"Hello Unicode" raw_string = r"C:\Program Files"
Null in Python and Java
Every language has a way of representing the absence of a value or a null value. In Java, this is represented by the keyword null
.
javaString str = null;
The variable str now does not hold any value and is considered null. This keyword can be assigned to any variable of reference types (classes, interfaces, arrays), but not to the primitive data types (int, float, etc.). On the contrary, Python treats None
as a unique instance of its data type (NoneType
), and it can be assigned to any variable.
pythonstr = None
Note that unlike Java's null
, Python's None
is a singleton, meaning there is always only one instance of None
in each Python program.
Lists in Python and Arrays in Java
In Java, arrays are a way to store multiple values of the same type. Meanwhile, Python uses lists, which can contain different types of objects.
Java arrays are fixed in size, and they don't offer built-in methods for manipulation:
javaint[] myArray = new int[5]; // Declare an array of integers
Python lists, however, are flexible and can easily grow or shrink. They also come with a variety of built-in methods for simple manipulation:
pythonmy_list = [1, "two", True]
In Python, immutability can be achieved using tuples, which are very similar to lists but cannot be changed once created:
pythonmy_tuple = (1, "two", 3.0)
HashMaps in Python and Java
HashMaps stores data in key-value pairs. Java uses HashMaps, while Python uses dictionaries (dict).
In Java, HashMaps are part of the collections framework:
javaHashMap<String, String> myHashMap = new HashMap<String, String>(); myHashMap.put("key1", "value1"); myHashMap.put("key2", "value2");
Python dictionaries are similar to Java's HashMaps but offer more flexibility with key types. Python dictionary keys can be any hashable type:
pythonmy_dict = { 'key1': 'value1', 2: 'value2', (3, 4): 'value3' }
Looping Structures
Java allows several types of loops such as for
, foreach
, while
, etc. For example, a for
loop in Java is written like this:
javafor (int i = 0; i < 5; i++) { System.out.println(i); }
In Python, loops are typically used to iterate over items of a sequence or iterator. The Python equivalent of the above Java for
loop is:
pythonfor i in range(5): print(i)
Python's range(5)
is similar to i < 5
in Java and generates numbers from 0 to 4.
Function Definition
In Java, functions are defined using the void
, access modifier public
, private
, default
, or protected
, and function names. Functions (methods) can be defined inside a class only.
javapublic class MyClass { public void myFunction(){ // Function body } }
Python, on the other hand, uses the def
keyword for function definitions:
pythondef my_function(): # Function body goes here pass
Unlike Java, Python supports defining functions outside of classes.
Function Arguments in Python and Java
Function arguments in programming languages define how we pass data to functions. Java and Python manage function arguments quite differently, each with its own set of rules and capabilities.
In Java, methods are specific about the arguments that they expect. If a function is called with fewer or more arguments than expected, a compile-time error occurs.
Java implements the concept of Method Overloading for handling a variable number of arguments, where multiple methods can have the same name with different parameters. As long as the compiler can differentiate between the methods based on their parameters, these functions can coexist.
javavoid myMethod(int a) { System.out.println(a); } void myMethod(int a, int b) { System.out.println(a); System.out.println(b); } myMethod(10); // Logs 10
For a variable number of arguments, Java uses varargs. You can pass multiple values by separating them with a comma, and they're accessible as an array within the method.
javavoid myMethod(int... nums) { for (int num : nums) { System.out.println(num); } } myMethod(10, 20); // Logs 10, 20
Python functions do not natively support method or function overloading as Java does. In Python, if we define a method multiple times with the same name but different parameters, the last one defined will overwrite all the previous definitions.
Calling a function with the wrong number of arguments results in a runtime error. Additionally, arguments can be made strict using optional type hinting
, introduced in Python 3.5. However, Python provides more explicit ways to handle a variable number of arguments and keyword arguments.
- Optional Keyword Parameters: Python functions can have optional keyword parameters by providing default values.
python
def foo(arg, use_thing=True, default_width=1): # Function body
- Arbitrary Number of Positional Parameters: Using an asterisk
*
before a parameter allows the function to accept any number of positional arguments, which are accessible as a tuple.pythondef foo(*args): # args is a tuple of all positional arguments passed
- Arbitrary Number of Keyword Parameters: Prefixing a parameter with two asterisks **
**
** enables the function to accept an arbitrary number of keyword arguments, available as a dictionary.pythondef foo(**kwargs): # kwargs is a dictionary of all keyword arguments passed
Python's systematic approach with *args
and **kwargs
provides a structured way to handle variable numbers of arguments, making the functions more readable and maintainable.
Python Specific Concepts
Python Decorators
Python decorators are a powerful and expressive feature that allows you to modify or enhance the behavior of functions or methods without changing their code. This concept does not have a direct counterpart in Java, making it a unique feature for Java programmers to learn.
Basic Decorator Syntax
A decorator in Python is a function that takes another function as an argument and extends its behavior without explicitly modifying it. Decorators are defined with the @
symbol followed by the decorator name.
Here's a simple example:
pythondef my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello!") say_hello()
This code will output:
vbnetSomething is happening before the function is called. Hello! Something is happening after the function is called.
Decorators can be used for logging, enforcing access control, instrumentation, and more. They allow for cleaner and more readable code, adhering to the "Don't Repeat Yourself" (DRY) principle.
You may be confused with the @
annotations used in Java. For example, @Override
is an annotation in Java that informs the compiler that the child class method is intended to override the method in its parent class (inheritance relationship). It doesn't change the behavior of the method it's annotating but instead acts as a compile-time check.
- Java Annotations are mainly used to give the Java compiler some additional information about your program. This information can also be used by runtime libraries or external tooling.
- Python Decorators, on the other hand, are used to modify the behavior of a function, method, or class. They're not just metadata but are executable code that wraps the decorated function.
List Comprehension
List comprehension is a unique feature in Python, enabling the efficient creation of lists. It's a more potent alternative to Java's for loop + list add approach.
Java:
javaList<Integer> squares = new ArrayList<>(); for (int i = 0; i < 10; i++) { squares.add(i * i); }
Python:
pythonsquares = [i**2 for i in range(10)]
Python Generators
Python generators are a powerful tool that allows you to create an iterator in a fast, memory-efficient manner.
Generators are defined like regular functions but use the yield
statement whenever they want to return data. Each time yield
is called, the state of the generator is "frozen", and the value is emitted. When the generator is iterated again, it resumes from where it left off.
pythondef simple_generator(): yield 1 yield 2 yield 3 for value in simple_generator(): print(value)
This will output 1, 2, and 3, each on a new line.
Generators are memory-efficient because they only produce one item at a time, perfect for reading large files, processing streams of data, or generating infinite sequences.
This guide provides an extensive comparison of Python language features for Java programmers. It's essential to remember that each language has its strengths and is more suited to specific types of tasks. A versatile programmer knows which language to use when!