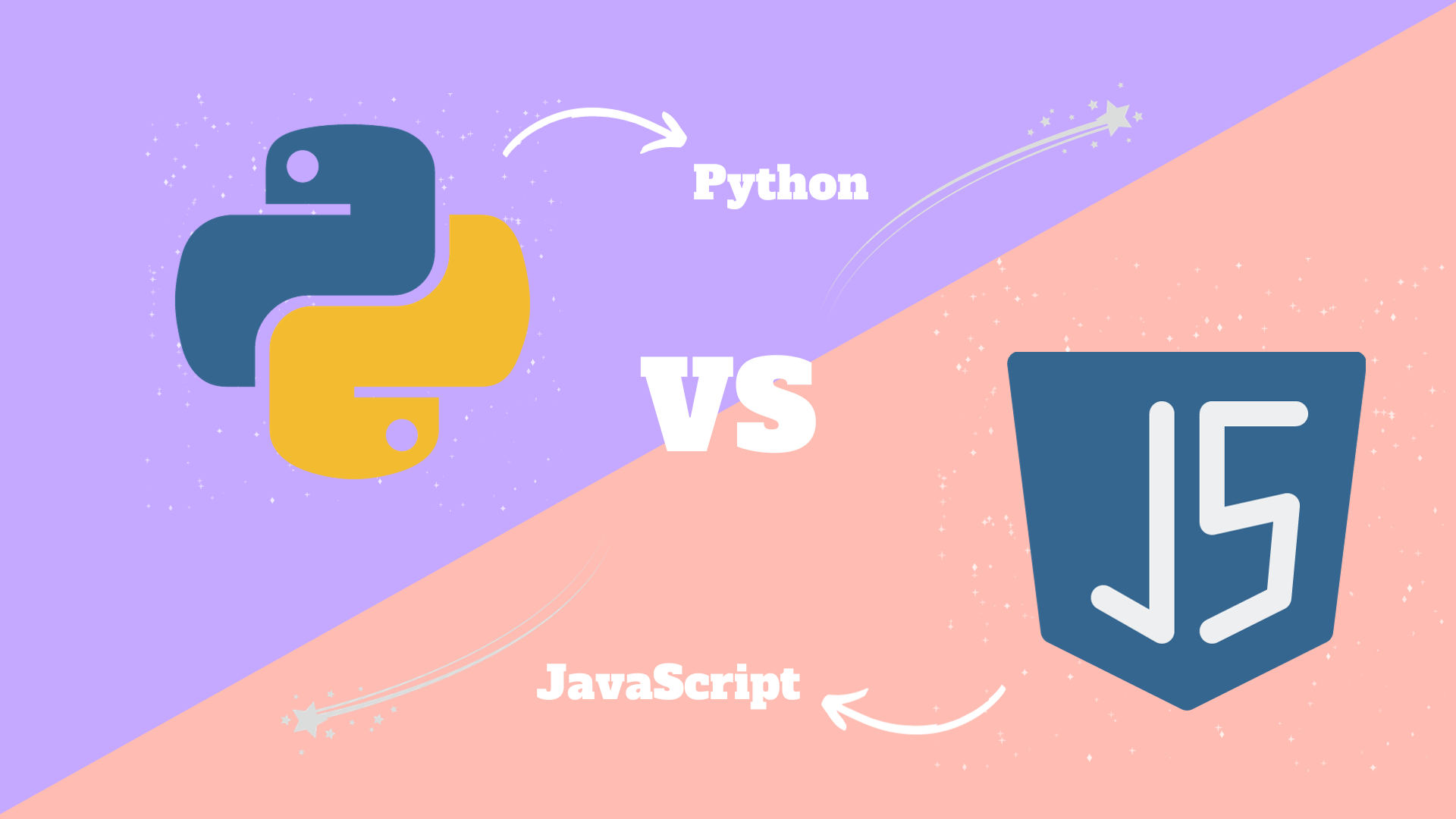
CONTENTS
Variable Declaration
Data Types in Python and JavaScript
Logical Variables
String
Nothingness in Python and JavaScript
List Type
HashMap Type in Python and JavaScript
Looping structures
Function Definition
Function Arguments in Python and JavaScript
Statement Terminators
Python Decorators
List Comprehension
Python Generator Functions
Are you a JavaScript programmer interested in learning Python? You're in the right place! This blog makes learning Python easy and fun, especially if you already know JavaScript. We will use simple words and clear examples. Our goal is to help you understand Python quickly and easily. Whether you want to add Python to your skills or solve specific problems, we've got you covered with straightforward and practical information.
Variable Declaration
In JavaScript, variables can be declared using var
or let
. The var
keyword has been around since the early days of JavaScript and declares a variable with function scope. On the other hand, let
, introduced in ES6, allows for block-scoped variable declaration, which can help prevent common bugs related to scoping.
Example in JavaScript:
jsxvar oldVariable = "I have function scope"; let newVariable = "I have block scope";
In JavaScript, it's also possible to declare a variable without immediately assigning a value to it. This variable will be undefined
until a value is assigned.
jsxvar emptyVar; console.log(emptyVar); // Output: undefined
Python handles variable declaration differently. There's no need for keywords like var
or let
. You simply declare a variable by assigning a value to it. This approach streamlines variable declaration and reduces the syntax overhead.
Example in Python:
pythonmy_variable = "I am a Python variable"
In Python, declaring a variable without assigning a value is not possible in the same way as JavaScript. Attempting to do so will result in a syntax error or unintended behavior.
Key Differences
- Declaration Keywords: JavaScript uses
var
andlet
(orconst
for constants) for variable declaration, while Python doesn't require any keyword. - Scoping Rules: JavaScript's
var
has function scope, andlet
has block scope. In Python, variables have a scope determined by where they are defined, such as inside a function or globally. - Undefined Variables: In JavaScript, a declared but unassigned variable will be
undefined
. Python, however, doesn't allow a variable to exist without an initial value.
Data Types in Python and JavaScript
When transitioning from JavaScript to Python, it's crucial to understand the similarities and differences in basic data types. This knowledge forms the foundation of your programming skills in Python. Let's delve into two primary data types: logical variables (booleans) and strings.
Logical Variables
Logical variables, commonly known as booleans, are a fundamental data type in most programming languages, including JavaScript and Python. They represent truth values and are often used in conditions and loops.
In JavaScript, the boolean values are true
and false
. These are lowercase and are part of the language's primitive types.
jsxlet isJavaScriptFun = true; let isPythonEasy = false;
Python, while similar in concept, differs slightly in syntax. The boolean values are True
and False
. Notice the capitalization of the first letter, which is a key difference from JavaScript.
pythonis_python_fun = True is_javascript_easy = False
This difference might seem minor, but it's crucial for avoiding syntax errors when switching between the two languages.
String
Strings, sequences of characters used for storing and manipulating text, are common in both JavaScript and Python. They can be enclosed in either single quotes ('
) or double quotes ("
), with no difference in their interpretation in both languages.
In JavaScript, strings are sequences of Unicode characters. This means that JavaScript strings can store any character from the Unicode standard.
jsxlet hello = "Hello World"; let greet = "Hello again!";
Python treats strings similarly. In Python 3 and above, a bare quoted string is a sequence of Unicode characters, just like in JavaScript. However, Python also allows for byte strings, which are sequences of bytes. These can be defined by prefixing the string with b
.
pythonnormal_string = "Hello Python" unicode_string = u"Hello Unicode" byte_string = b"Hello Bytes"
In Python, the u
prefix is optional in version 3 and above, as strings are Unicode by default. However, the b
prefix is used to specifically create a byte string, which behaves differently from a Unicode string.
Nothingness in Python and JavaScript
Understanding how a programming language represents 'nothingness' or the absence of a value is crucial. In JavaScript, this concept is represented by two values: undefined
and null
. Python, on the other hand, has a single representation: None
.
In JavaScript, undefined
and null
are used to represent absence, but they have distinct uses.
-
Undefined: This value is automatically assigned to variables that have been declared but not yet assigned a value. Additionally, when you try to access an attribute of an object that does not exist, JavaScript returns
undefined
.jsxlet unassignedVariable; console.log(unassignedVariable); // undefined let obj = {}; console.log(obj.nonExistentProperty); // undefined
-
Null: The
null
value, in contrast, is used to explicitly denote a non-existent or empty value. It's often used when you want to intentionally clear or reset a variable.jsxlet emptyValue = null;
Python simplifies this concept by having only one representation of nothingness: None
.
-
None: In Python,
None
is used to denote absence or null values. Variables in Python cannot exist without being initialized; attempting to access an undeclared variable results in aNameError
. For dictionaries and object attributes, trying to access non-existent keys or attributes results in aKeyError
andAttributeError
, respectively.pythonnon_existent_variable = None # Explicitly setting to None # Accessing an undefined variable results in NameError # print(unknown_variable) # This would cause a NameError my_dict = {'key': 'value'} # Accessing a non-existent key in a dictionary # print(my_dict['non_existent_key']) # This would cause a KeyError
List Type
Both Python and JavaScript use lists (arrays in JavaScript) as a way to store sequences of items. These lists are versatile and can contain items of different data types.
In JavaScript, lists are known as arrays. They are declared using square brackets and can contain elements of different types.
jsxlet myArray = [1, "two", true];
Arrays in JavaScript are dynamic and can grow or shrink in size. They come with a variety of built-in methods for manipulation, like push
, pop
, slice
, and more.
Python also uses square brackets to declare lists, and like JavaScript, these lists can contain elements of different types.
pythonmy_list = [1, "two", True]
Python lists are similar to JavaScript arrays in terms of flexibility and available methods for manipulation. Additionally, Python offers a unique type called a tuple.
-
Tuple: Tuples are similar to lists but are immutable. Once a tuple is created, its elements cannot be changed. Tuples can be declared using parentheses, but these can also be omitted.
pythonmy_tuple = (1, "two", True) simple_tuple = 1, "two", True # Parentheses are optional
Tuples are useful in scenarios where a sequence of values should not be altered and offer slight performance improvements over lists due to their immutability.
HashMap Type in Python and JavaScript
HashMaps are data structures that store data in key-value pairs. In JavaScript, this is primarily accomplished using objects, while Python uses dictionaries (dict) and sets.
In JavaScript, objects are collections of properties, and they are declared using curly braces. Each property is a key-value pair, and the key is always converted to a string unless it's a symbol.
jsxlet var_key = "key4"; let myObject = { key1: "value1", key2: "value2", 3: "value3", [var_key]: "value4", }; console.log(Object.keys(myObject)); // ['key1', 'key2', '3']
Python's dictionaries (dict
) are similar but offer more flexibility with key types. In Python, dictionary keys can be any immutable, hashable type, not just strings.
pythonmy_dict = { 'key1': 'value1', 2: 'value2', (3, 4): 'value3' }
Python also has set
, which is a collection of unique elements, but it doesn't store key-value pairs like dictionaries
Looping structures
In JavaScript, you might write a for
loop like this:
jsxfor (let i = 0; i < 5; i++) { console.log(i);
In Python, the for
loop is typically used to iterate over items of a sequence (like a list) or an iterator. The syntax is simpler:
pythonfor i in range(5): print(i)
Note that range(5)
in Python is similar to i < 5
in JavaScript. It generates numbers from 0 to 4.
The while
loop in Python is quite similar to JavaScript. Here's a JavaScript while
loop:
jsxlet i = 0; while (i < 5) { console.log(i); i++; }
In Python, it looks very similar:
pythoni = 0 while i < 5: print(i) i += 1
Notice the absence of curly braces and semicolons in Python. Indentation is key here, as it defines the scope of the loop.
Loop Control Statements
Both JavaScript and Python use break
to exit a loop and continue
to skip the current iteration and proceed to the next one.
For-Else Loop (Python Specific)
Python offers a unique for-else
loop, where the else
block runs after the loop completes normally, but not when the loop is exited with a break
statement. This feature doesn't exist in JavaScript.
Example:
pythonfor i in range(5): print(i) else: print("Completed without break")
Function Definition
The way functions are defined and used in Python and JavaScript varies significantly, especially considering the flexibility and syntax.
In JavaScript, the function
keyword is used to define functions. Functions can be named or anonymous. JavaScript also supports arrow functions, which provide a more concise syntax and lexical scoping of this
.
jsxfunction namedFunction() { // ... } let anonymousFunction = function () { // ... }; let arrowFunction = () => { // ... };
Python uses the def
keyword for function definitions. Unlike JavaScript, Python functions must have a name when defined with def
.
pythondef my_function(): # ...
For anonymous functions, Python provides lambda
expressions. However, lambdas in Python are more limited compared to JavaScript's anonymous functions. Lambdas in Python can only contain expressions, not statements, and they cannot contain complex control structures like if
blocks, loops, or multiple return points.
pythonlambda_function = lambda x: x * 2
While JavaScript's anonymous functions can be as complex as named functions, Python's lambdas are best suited for simple, single-expression functions and often used in contexts like arguments to higher-order functions.
Function Arguments in Python and JavaScript
Function arguments in programming languages define how we pass data to functions. JavaScript and Python handle function arguments quite differently, each with its own set of rules and capabilities.
In JavaScript, functions are very flexible with the arguments passed to them. If a function is called with fewer arguments than expected, the missing arguments are assigned the value undefined
. If extra arguments are passed, they are accessible via the arguments
object, which is available within each function scope.
jsxfunction myFunction(a, b) { console.log(a); // First argument console.log(b); // Second argument or undefined if not provided console.log(arguments.length); // Number of arguments passed } myFunction(10); // Logs 10, undefined, 1
The arguments
object is array-like but not an actual array. It doesn't have array methods like pop
, but it allows you to access arguments by their index and determine how many arguments were passed.
Python functions, in contrast, are more strict. Calling a function with the wrong number of arguments results in an exception. However, Python provides more explicit ways to handle variable numbers of arguments and keyword arguments.
- Optional Keyword Parameters: Python functions can have optional keyword parameters by providing default values.
python
def foo(arg, use_thing=True, default_width=1): # Function body
- Arbitrary Number of Positional Parameters: Using an asterisk `` before a parameter allows the function to accept any number of positional arguments, which are accessible as a tuple.
python
def foo(*args): # args is a tuple of all positional arguments passed
- Arbitrary Number of Keyword Parameters: Prefixing a parameter with two asterisks
*
enables the function to accept an arbitrary number of keyword arguments, available as a dictionary.pythondef foo(**kwargs): # kwargs is a dictionary of all keyword arguments passed
Python's approach with *args
and **kwargs
offers a structured way to handle variable numbers of arguments, making the functions more readable and maintainable.
It's important to note that the behavior of keyword parameters and arbitrary numbers of parameters in Python has evolved, particularly with the introduction of Python 3. For detailed changes, one should refer to the Python Enhancement Proposals (PEPs) relevant to these features.
While both JavaScript and Python can achieve similar patterns and flexibility with function arguments, their approaches differ. JavaScript relies on the arguments
object for flexibility, whereas Python uses explicit syntax with *
and **
to achieve similar functionality.
Statement Terminators
In JavaScript, semicolons are used to terminate statements. While they are technically optional due to JavaScript's Automatic Semicolon Insertion (ASI), relying on ASI can sometimes lead to unexpected issues, although these cases are rare.
jsxlet a = 3; let b = 4;
In contrast, Python uses a newline to mark the end of a statement, making code more readable and succinct. Semicolons are not required at the end of each line.
pythona = 3 b = 4
Python does allow the use of semicolons to separate multiple statements on a single line. However, this is generally discouraged as it goes against Python's design philosophy of readability and simplicity.
pythona = 3; b = 4 # Not recommended in Python
Python’s approach encourages cleaner and more readable code, aligning with its philosophy of simplicity and explicitness.
Python Decorators
Python decorators are a powerful and expressive feature that allows you to modify or enhance the behavior of functions or methods without changing their code. This concept does not have a direct counterpart in JavaScript, making it a unique feature for JavaScript programmers to learn.
Basic Decorator Syntax
A decorator in Python is a function that takes another function as an argument and extends its behavior without explicitly modifying it. Decorators are defined with the @
symbol followed by the decorator name.
Here's a simple example:
pythondef my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello!") say_hello()
This code will output:
vbnetSomething is happening before the function is called. Hello! Something is happening after the function is called.
Decorators can be used for logging, enforcing access control, instrumentation, and more. They allow for cleaner and more readable code, adhering to the "Don't Repeat Yourself" (DRY) principle.
List Comprehension
List comprehension is a distinctive feature in Python, allowing for concise and efficient creation of lists. It's a form of syntactic sugar that can perform the equivalent of JavaScript's map
and filter
methods in a more elegant way.
A basic list comprehension in Python involves an expression followed by a for
clause, and optionally, if
clauses. The expression can be any expression that evaluates to a value and can include function calls.
Example:
pythonsquares = [x**2 for x in range(10)]
This creates a list of squares of numbers from 0 to 9.
In JavaScript, you might use map
to apply a function to each item in an array:
jsxconst numbers = [1, 2, 3, 4, 5]; const squares = numbers.map((x) => x * x);
The Python equivalent using list comprehension is more direct:
pythonnumbers = [1, 2, 3, 4, 5] squares = [x * x for x in numbers]
Equivalent of JavaScript's filter
Similarly, Python's list comprehension can elegantly replicate JavaScript's filter
functionality:
JavaScript version:
jsxconst numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter((x) => x % 2 === 0);
Python version:
pythonnumbers = [1, 2, 3, 4, 5] evenNumbers = [x for x in numbers if x % 2 == 0]
Python Generator Functions
Python generator functions are a simple way to create iterators. They allow you to declare a function that behaves like an iterator, i.e., it can be used in a for
loop.
Generators are defined like regular functions but use the yield
statement whenever they want to return data. Each time yield
is called, the state of the generator is "frozen," and the value is emitted. When the generator is iterated again, it resumes from where it left off.
Example:
pythondef my_generator(): yield 1 yield 2 yield 3 for value in my_generator(): print(value)
This will output 1, 2, and 3, each on a new line.
Generators are memory-efficient because they only produce one item at a time, perfect for reading large files, processing streams of data, or generating infinite sequences.